.NET Microsoft Entity Framework - Code First Example
After adding the CipherDB SDK to your application using NuGet, add one line of code to your database context constructor to open a CipherDB enabled session.
public class Context: DbContext
{
public Context(): base()
{
}
public DbSet<SampleEntity> SampleEntities{ get; set; }
}
public class Context: DbContext
{
public Context(): base()
{
Crypteron.CipherDb.Session.Open(this);
}
public DbSet<SampleEntity> SampleEntities{ get; set; }
}
Add the attribute [Secure]
to any properties of your entity that you want to encrypt.
public class SampleEntity
{
public SampleEntity()
{
}
public int SampleEntityId {get; set;}
public string Description {get; set;}
public string CreditCardNumber {get; set;}
public string SocialSecurityNumber {get; set;}
}
public class SampleEntity
{
public SampleEntity()
{
}
public int SampleEntityId {get; set;}
public string Description {get; set;}
[Secure]
public string CreditCardNumber {get; set;}
[Secure]
public string SocialSecurityNumber {get; set;}
}
NOTE: A database-first approach is supported as well. See the Documentation for details.
Java Persistence API – Hibernate Example
After installing the CipherDB SDK to your application using Maven, add the annotation @Secure
to any properties of your entity that you want to encrypt.
public class SampleEntity
{
public SampleEntity()
{
}
@Id
@Column(name="SampleEntityId")
private int SampleEntityId;
@Column(name="Description")
private String Description;
@Column(name="CreditCardNumber")
private String CreditCardNumber;
@Column(name="SocialSecurityNumber")
private String SocialSecurityNumber;
}
public class SampleEntity
{
public SampleEntity()
{
}
@Id
@Column(name="SampleEntityId")
private int SampleEntityId;
@Column(name="Description")
private String Description;
@Secure
@Column(name="CreditCardNumber")
private String CreditCardNumber;
@Secure
@Column(name="SocialSecurityNumber")
private String SocialSecurityNumber;
}
And that’s it! Your sensitive data is automatically signed and encrypted before being transmitted or persisted. The data is then decrypted on the way back so the rest of your application can function normally without worrying about data security. It’s that easy!
See It In Action (2 Minute Video)
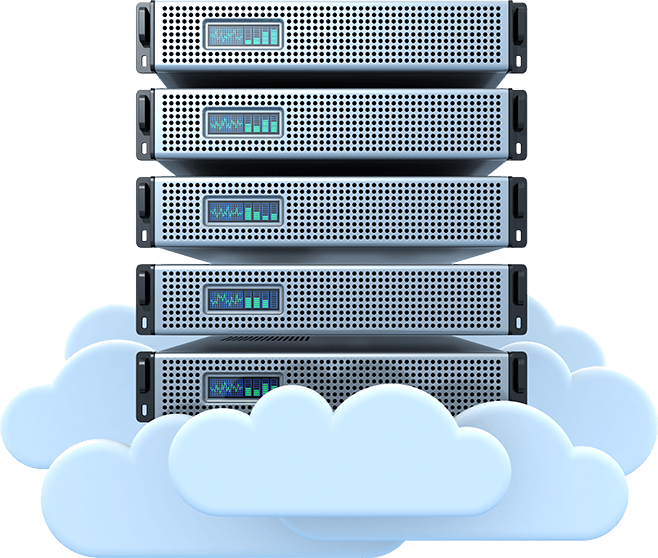
CipherDB in the Cloud
Despite their excellent infrastructure, major cloud databases like Amazon RDS and Microsoft Azure SQL do NOT provide robust data-at-rest security. Traditional database encryption stores data and encryption keys on the same server, right next to each other! Features like SQL Transparent Data Encryption (TDE) do not protect your data from those who gain access to the database. Finally, since you do not own or maintain the database servers, the cloud providers will always have administrative access to your data.
The bottom line is that data stored in the cloud is inherently insecure. In order to achieve true data security, you need to encrypt your sensitive data at the source before it ever leaves your server application.
Surveys and reports all point out that the #1 reason why companies cannot benefit from cloud computing is because of security and privacy concerns, especially when dealing with sensitive data such as medical records or financial information.
Don’t let your organization miss out on the cloud computing revolution! With CipherDB you’re in control of your data, regardless of where it’s stored. The encryption keys are stored on a separate server and encrypted themselves with elliptic curve cryptography.
CipherDB works with any cloud provider including
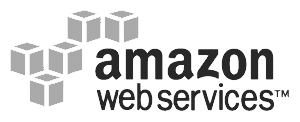
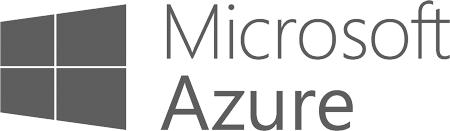
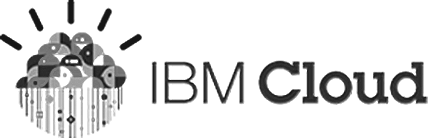
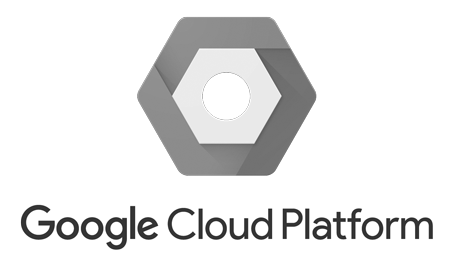
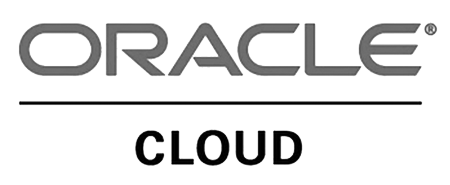
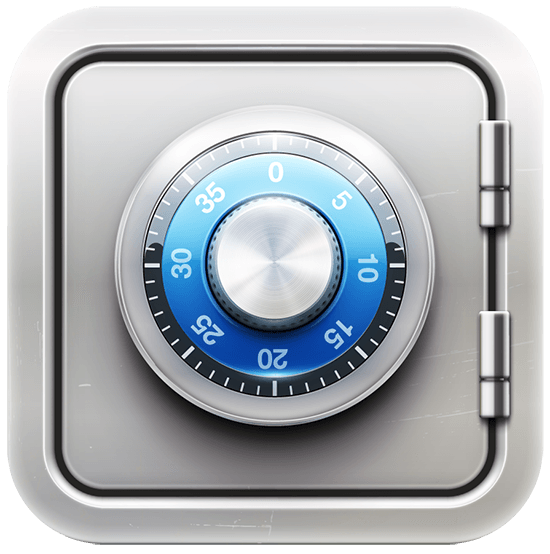
CipherDB on Premises
For on-premise or hybrid applications, CipherDB provides multiple layers of encryption that are essential for defense-in-depth and secure-by-designs principals through application layer encryption.
Lower maintenance and higher reliability will attract more and more organizations towards a pure public cloud solution. In the meantime, CipherDB’s strong encryption and automatic key management helps out in almost every situation
Key Management and more with Crypteron
CipherDB integrates with Crypteron’s security platform which handles key management, multiple security partitions, access control rules, auditing, key rotations, key migrations and more. Crypteron encrypts your encryption keys and stores them separately from the application and your data, so your data is always secure.
Crypteron’s security platform includes an easy-to-use dashboard at my.crypteron.com.
View all of your Apps
Each App can be optionally divided into multiple Security Partitions
Each Security Partition has an Access Control List where you can specify roles and permissions
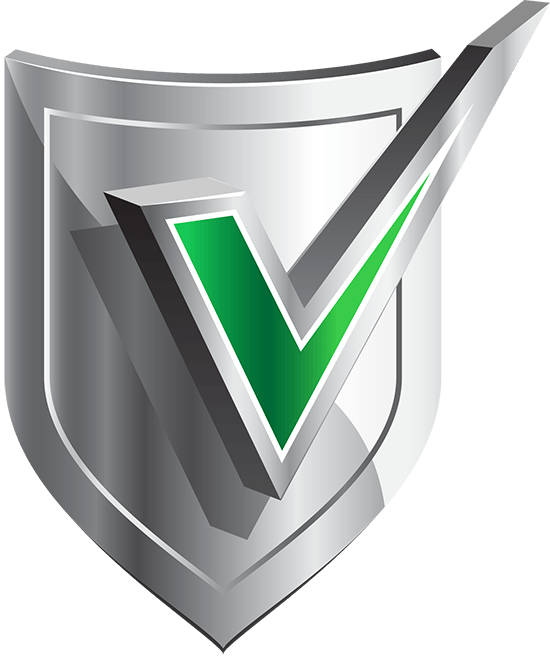
Compliance in the Cloud
CipherDB makes it possible to deploy your application to the public cloud while also complying with your organization’s security policy or 3rd party standards such as HIPAA, PCI, FIPS-140-2, CJIS / Law Enforcement, FISMA, EU Data Protection and more.
HIPAA Compliance
CipherDB satisfies sections of the Security Standards and Safeguards under HIPAA. Security and privacy of patient medical records is impossible to achieve in the cloud paradigm without encryption. If you properly encrypt your data and a data breach takes place, you are not liable for negligence
PCI Compliance
Any company that touches credit card information, must secure that data in order to be PCI compliant. If the data is stored in a database, it must be encrypted within the storage medium and the encryption keys must be properly managed. Failure to do so cause the vendor to be stripped of its ability to accept credit cards and be subject to hefty fines.
CipherDB Makes Compliance Simple
In order to be compliant, most organizations either abandon the public cloud or build their own encryption infrastructure which takes a tremendous amount of time, resources, and technical expertise. CipherDB provides the best of both worlds, allowing you to take advantage of the cloud while taking care of the entire process of encryption, key management and key migrations. Your team can now focus on your core business and not waste time and resources solving a problem already solved and solved well.
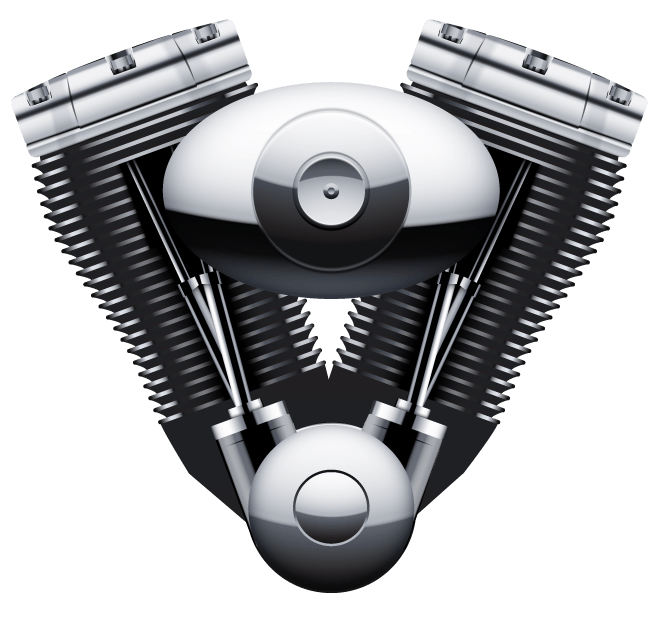
Under the Hood
Briefly stated, CipherDB uses FIPS-140-2 certified elliptic curve cryptography and AES 256 bit in the latest GCM mode to protect your data. The encryption is so robust that even the National Security Agency (NSA) recommends it for the protection of government and military classified information. In fact AES256-GCM is even permitted to encrypt sensitive data classified as “TOP-SECRET” – the highest classification level possible. CipherDB’s use of 521-bit elliptic curve cryptography is stronger than 15,000-bit RSA. With CipherDB you get that level of encryption with marginal overhead – just about 1 ms per record containing multiple encrypted fields. Plus database operations usually travel over a network, which means the real world performance is almost the same as using no encryption at all. The technical specification document can be found here. More details can be found in our developers guide.